There are many situations when a
user wants to allow a user to upload more than one file, you're stuck with
either adding as many file input elements as the number of files you want to
upload, or possibly having new ones appear 'magically' through JavaScript.
This article helps in uploading
multiple files simultaneously in ASP.Net . This article uses HTML File Input
Control and Grid View.
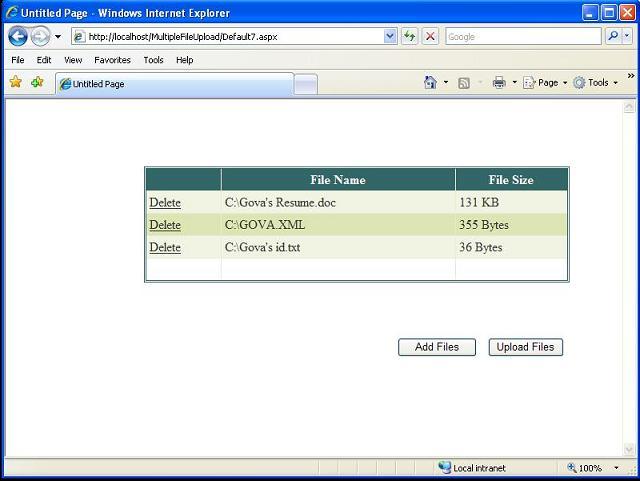
Using this article doesn't get much simpler. Thanks to
Microsoft's DotNet.
The heart of this article is the script which helps to
get the FileOpen Dialog in ASP.Net
<script type="text/javascript" language="javascript">
function getFile()
{
document.getElementById("file1").click();
var file = "";
if(document.getElementById("TextBox1").value == "")
file = document.getElementById("file1").value;
else
file = document.getElementById("TextBox1").value + "\r\n" + document.getElementById("file1").value;
document.getElementById("TextBox1").value = file ;
}
</script>
To use this article. just run the web application, once
done its easy just click the Add Files Button to start uploading the files the
file along with the details is shown in the Grid View.
The Code for the Add Files Button Click is shown below :
protected void Button1_Click(object sender, EventArgs e)
{
if (TextBox1.Text.Length > 0)
{
DataTable dt;
DataRow dr = null;
FileInfo fileObj = new FileInfo(TextBox1.Text.Trim());
long size = fileObj.Length / 1024;
loggedUser = "Administrator";
folderPath = System.Web.Hosting.HostingEnvironment.MapPath("~/" + loggedUser);
System.IO.DirectoryInfo dirObj = new DirectoryInfo(folderPath);
if (!dirObj.Exists)
dirObj.Create();
try
{
fileObj.CopyTo(folderPath + "\\" + fileObj.Name);
}
catch (Exception ee)
{
TextBox1.Text = "";
string error = ee.Message.ToString();
Response.Write("<script> window.alert(' File With the same name already uploaded ')</script>");
return;
}
if (GridView1.Rows.Count == 0)
{
dt = new DataTable();
DataColumn dc1 = new DataColumn("File Name", typeof(string));
DataColumn dc2 = new DataColumn("File Size", typeof(string));
dt.Columns.Add(dc1);
dt.Columns.Add(dc2);
dr = dt.NewRow();
dr["File Name"] = TextBox1.Text.ToString().Trim();
if (size > 0)
dr["File Size"] = size.ToString() + " KB";
else
dr["File Size"] = fileObj.Length.ToString() + " Bytes";
dt.Rows.Add(dr);
GridView1.DataSource = dt;
GridView1.DataBind();
}
else
{
int count = GridView1.Rows.Count;
dt = new DataTable();
DataColumn dc1 = new DataColumn("File Name", typeof(string));
DataColumn dc2 = new DataColumn("File Size", typeof(string));
dt.Columns.Add(dc1);
dt.Columns.Add(dc2);
for (int i = 0; i < count; i++)
{
dr = dt.NewRow();
dr["File Name"] = GridView1.Rows[i].Cells[1].Text;
dr["File Size"] = GridView1.Rows[i].Cells[2].Text;
dt.Rows.Add(dr);
}
dr = dt.NewRow();
dr["File Name"] = TextBox1.Text.ToString().Trim();
if(size > 0)
dr["File Size"] = size.ToString() + " KB";
else
dr["File Size"] = fileObj.Length.ToString() + " Bytes";
dt.Rows.Add(dr);
GridView1.DataSource = dt;
GridView1.DataBind();
}
TextBox1.Text = "";
}
}
if u wish to delete the file then just use the delete
option in the Grid View.
Once all the files to be uploaded are added u can
continue processing the files and Upload the files by clicking the Upload
Files Button. The processing code for Upload Files Button is left to the user.
You can download the project at
http://www.codeproject.com/useritems/Multiple_File_Uploader.asp